By: CS2113T-W12-4
Since: Jun 2016
Licence: MIT
1. Setting up
1.1. Prerequisites
-
JDK
9
or laterJDK 10
on Windows will fail to run tests in headless mode due to a JavaFX bug. Windows developers are highly recommended to use JDK9
. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
1.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
1.3. Verifying the setup
-
Run the
seedu.address.MainApp
and try a few commands -
Run the tests to ensure they all pass.
1.4. Configurations to do before writing code
1.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
1.4.2. Updating documentation to match your fork
After forking the repo, the documentation will still have the SE-EDU branding and refer to the se-edu/addressbook-level4
repo.
If you plan to develop this fork as a separate product (i.e. instead of contributing to se-edu/addressbook-level4
), you should do the following:
-
Configure the site-wide documentation settings in
build.gradle
, such as thesite-name
, to suit your own project. -
Replace the URL in the attribute
repoURL
inDeveloperGuide.adoc
andUserGuide.adoc
with the URL of your fork.
1.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your personal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
1.4.4. Getting started with coding
When you are ready to start coding,
-
Get some sense of the overall design by reading Section 2.1, “Architecture”.
-
Take a look at [GetStartedProgramming].
2. Design
2.1. Architecture
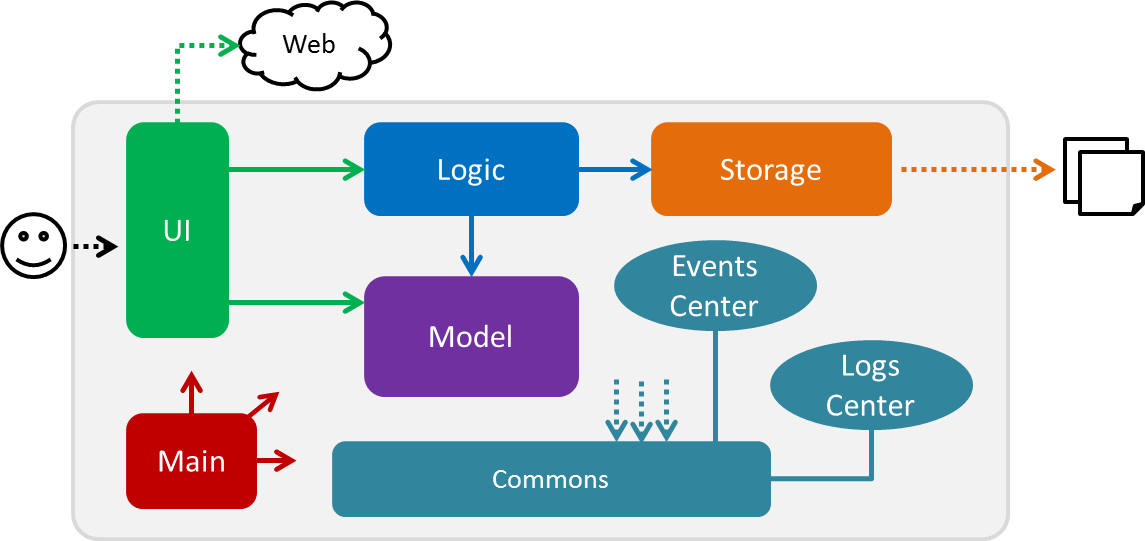
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
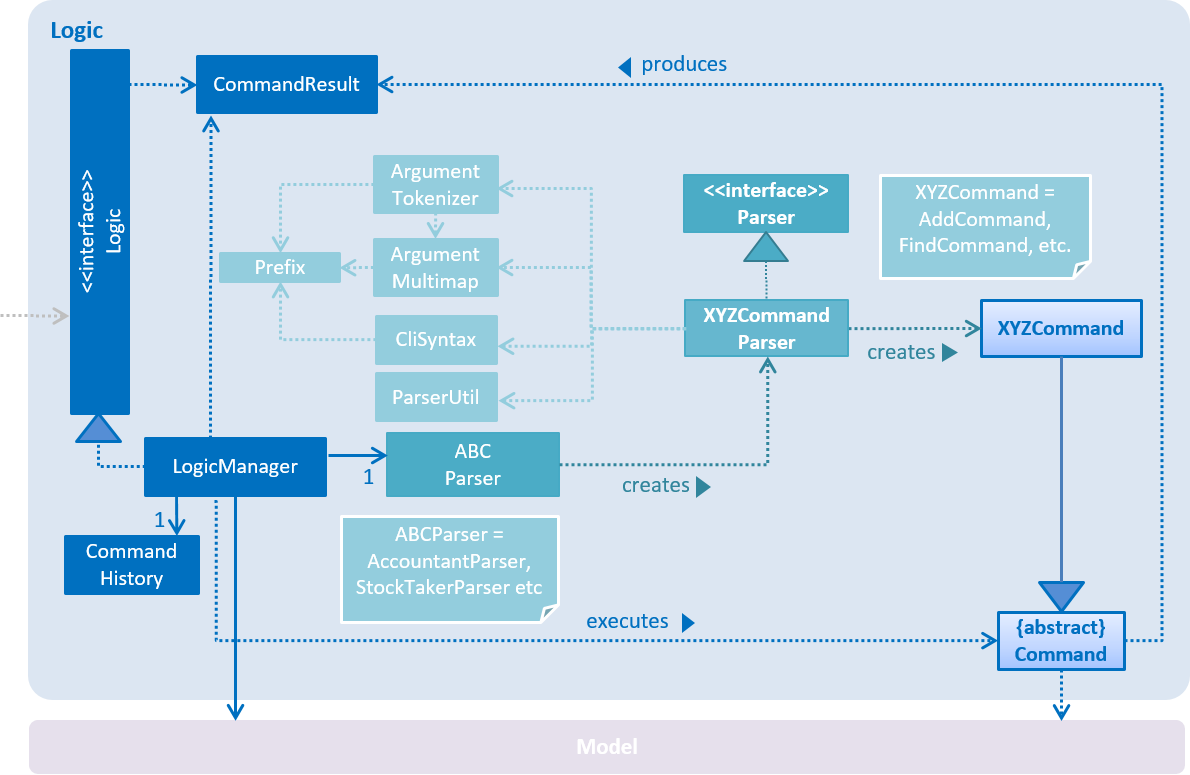
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command delete 1
.
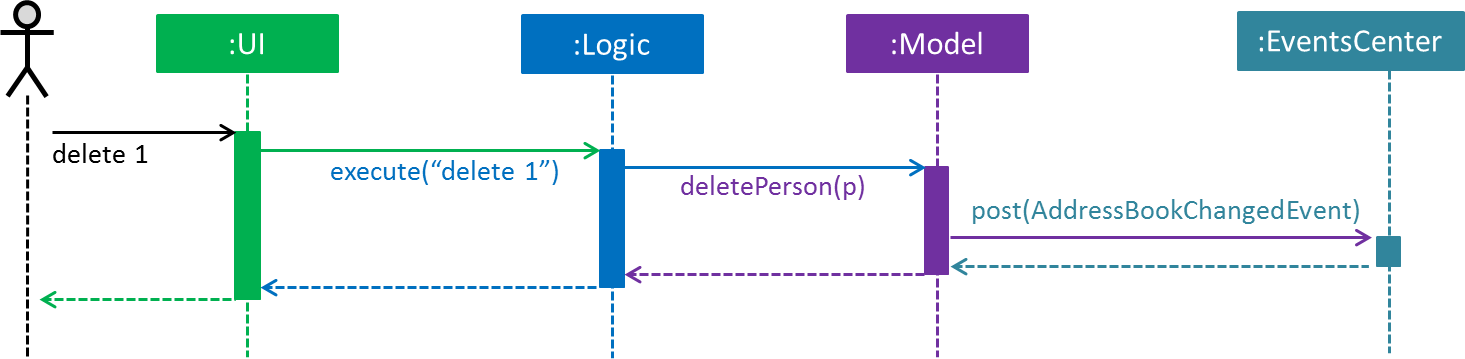
delete 1
command (part 1)
Note how the Model simply raises a AddressBookChangedEvent when the Address Book data are changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
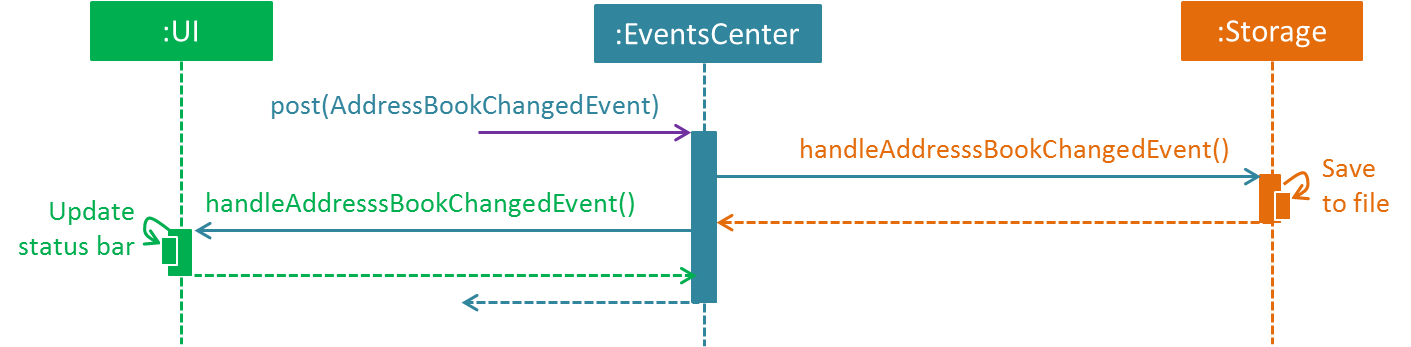
delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
2.2. UI component
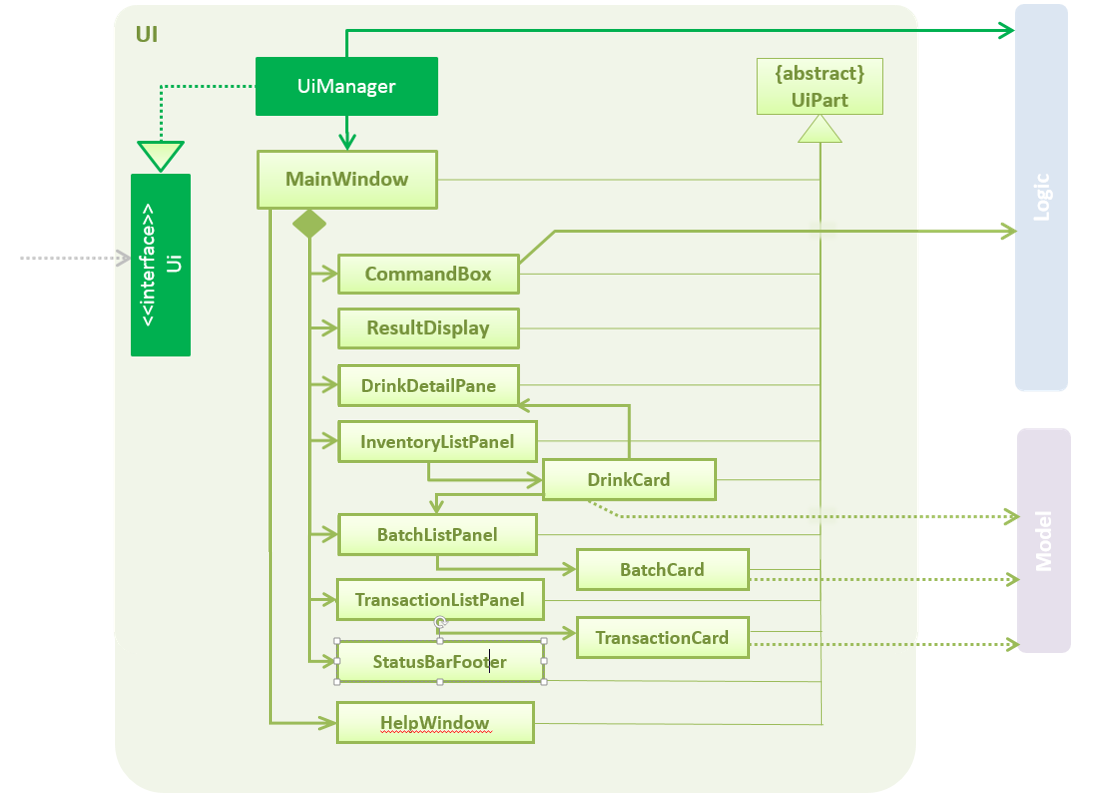
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, InventoryListPanel
, BatchListPanel
, StatusBarFooter
, DrinkDetailPane
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
2.3. Logic component
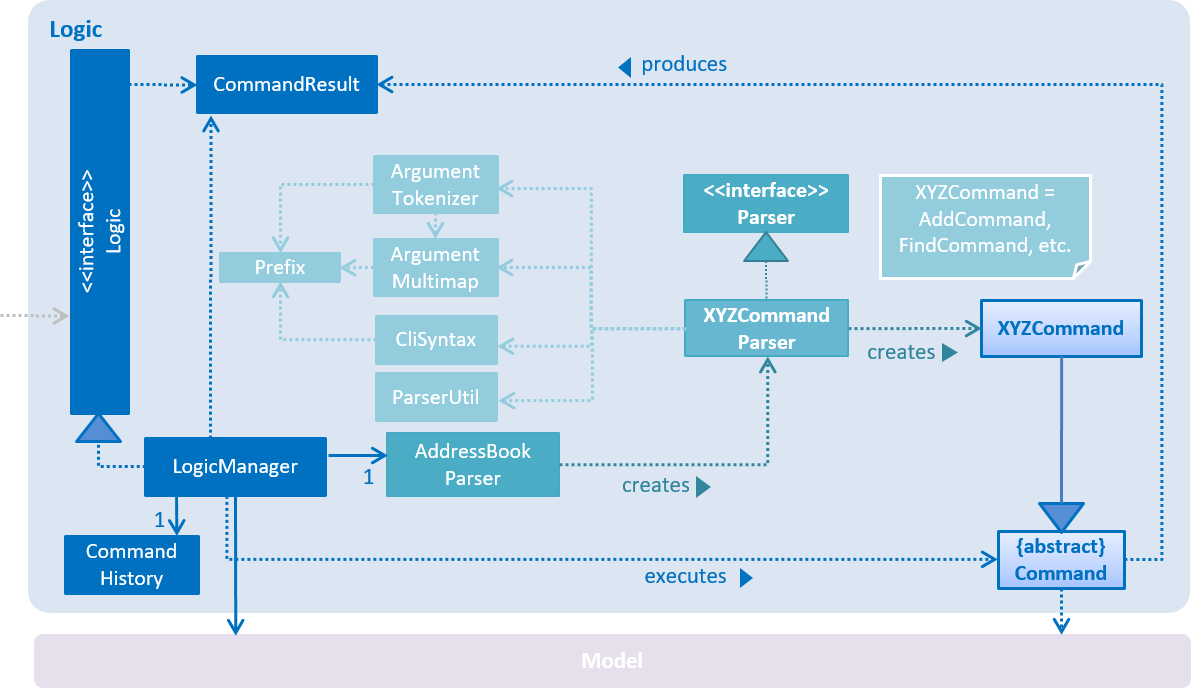
API :
Logic.java
-
Logic
uses theAddressBookParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a person) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
2.4. Model component
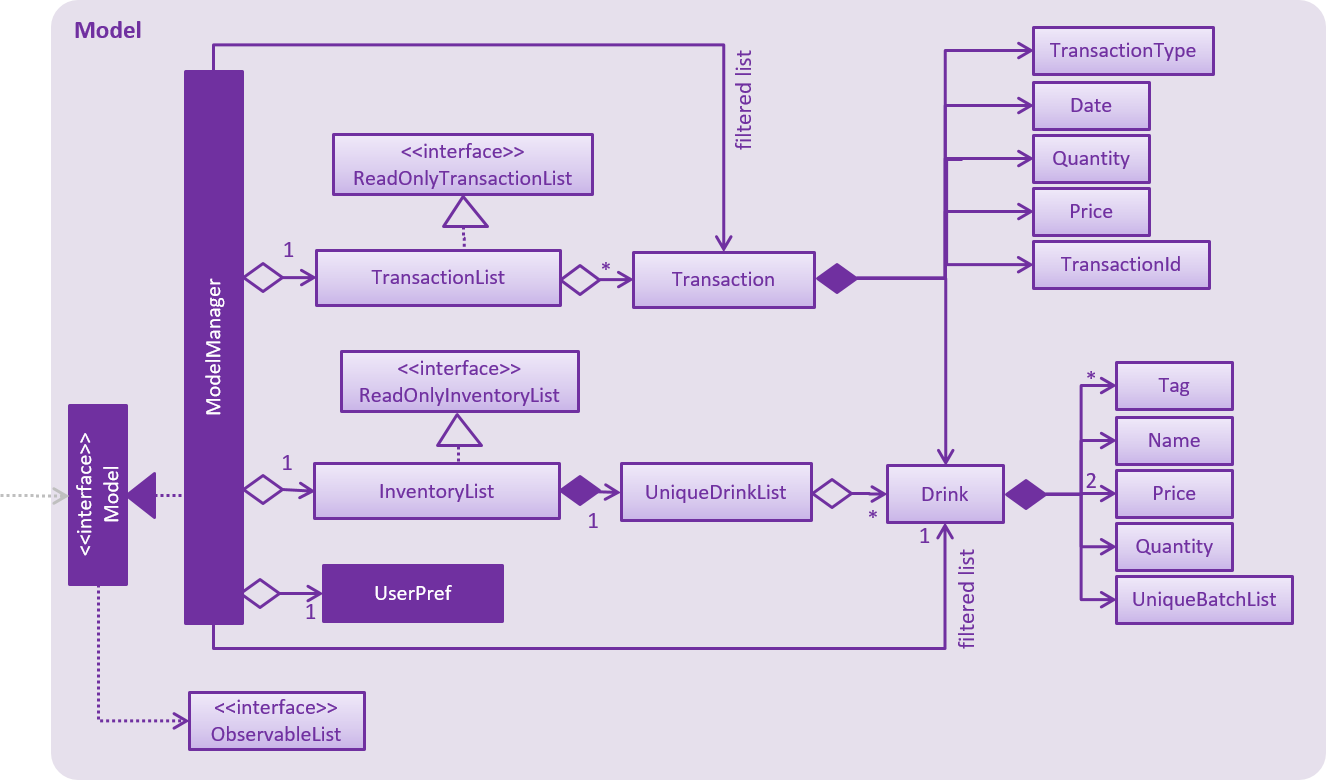
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the InventoryList data.
-
exposes an unmodifiable
ObservableList<Drink>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
stores the TransactionList data.
-
exposes an unmodifiable
ObservableList<Transaction>
that can be observed -
does not depend on any of the other three components.
2.5. Storage component
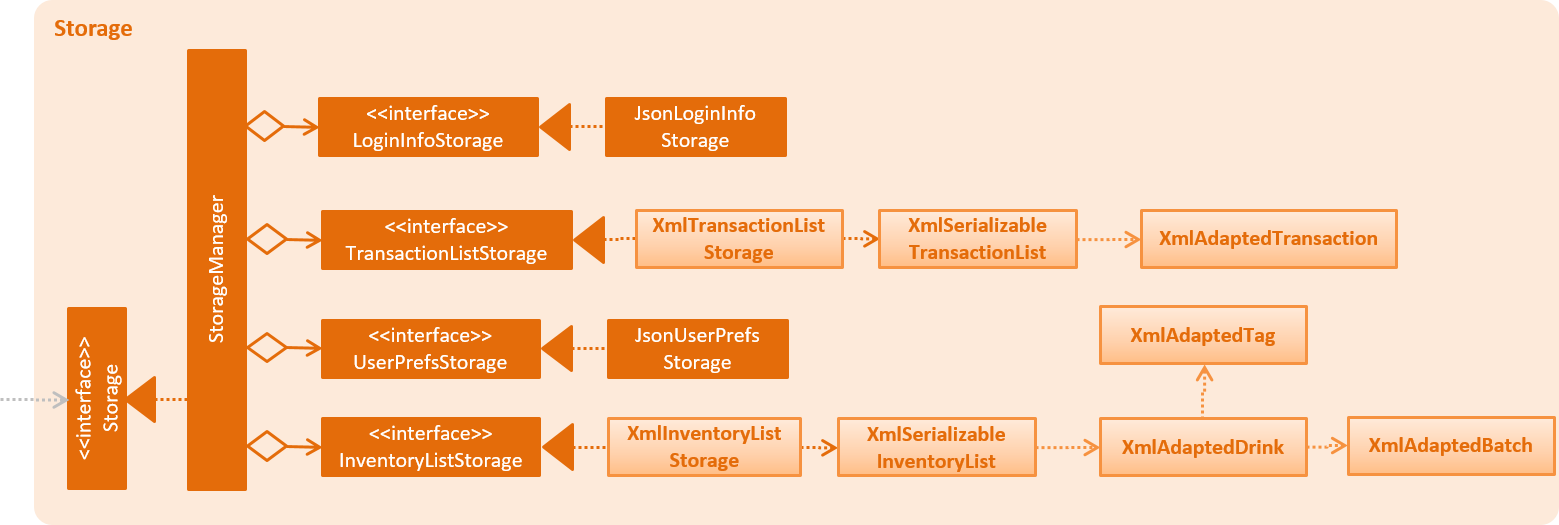
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read them back. -
can save the
LoginInfo
objects in json format and read them back. -
can save the Inventory List data in xml format and read it back.
-
can save the Transaction List data in xml format and read it back.
2.6. Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
3. Implementation
This section describes some noteworthy details on how certain features are implemented.
3.1. Analyses of costs, revenue and profit in the company over periods of time
3.1.1. Implementation
To facilitate the analyses of total profit, revenue and costs of the company over various periods of time,
transactions (sales and purchases) are logged using Transaction
s.
They will be logged when the drink stock increases due to a purchase (TransactionType
is PURCHASE
),
or when they decrease due to a sale (TransactionType
is SALE
).
These transactions are stored into a list of transactions, in TransactionList
.
Analyses are conducted in AnalysisManager
, with the API exposed in Analysis
.
The following operations are implemented for the analyses of profit, revenue and costs:
-
Analysis#analyseCost(AnalysisPeriodType period)
-
Analysis#analyseRevenue(AnalysisPeriodType period)
-
Analysis#analyseProfit(AnalysisPeriodType period)
The implementation of these operations are elaborated in the below sub-sections.
3.1.2. Indicating the analysis period with AnalysisPeriodType
AnalysisPeriodType
is an enum class that denotes the period for analysis:
-
in the current day
-
within the past 7 days (including the current day)
-
within the past 30 days (including the current day)
These are indicated respectively as:
-
AnalysisPeriodType.DAY
-
AnalysisPeriodType.WEEK
-
AnalysisPeriodType.MONTH
.
A user may enter an analysis command with additional time period parameters.
For the period of a day, no parameter is indicated.
For the period of 7 days, -w
is appended to the command. (e.g. costs -w
)
For the period of 30 days, -m
is appended to the command. (e.g. costs -m
)
The following is the sequence of steps when the user enters an analysis command with a time period:
Step 1. User enters an analysis command (e.g. costs -m
).
Step 2. The appropriate parser (e.g. AnalyseCostsCommandParser
) parses the time period parameter,
and retrieves the AnalysisPeriodType
value corresponding to the time period.
Step 3. The relevant TransactionPeriodPredicate
for the AnalysisPeriodType
value is retrieved. It is assigned to an Analysis Command (e.g. AnalyseCostsCommand
).
The available TransactionPeriodPredicate
s corresponding to each AnalysisPeriodType
are:
-
DAY
:TransactionInADayPredicate
-
WEEK
:TransactionInSevenDaysPredicate
-
MONTH
:TransactionInThirtyDaysPredicate
These are retrieved using AnalysisPeriodType#getPeriodFilterPredicate()
.
Step 4. The analysis command is executed, and the TransactionPeriodPredicate
predicate is passed to the appropriate ModelManager
.
This is done through the analyseCosts()
method in the appropriate ModelManager
.
Step 5. In the appropriate ModelManager
(Accountant or Manager), the predicate is passed to the filtered transaction list.
This is in order to filter the transactions by their dates such that
the filtered transaction list only contains transactions with dates within the relevant period.
FilteredList<Transaction>
handles the filtering using the given predicate.
The following sequence diagram shows how the first part of the AnalyseCostCommand
works, as described above:
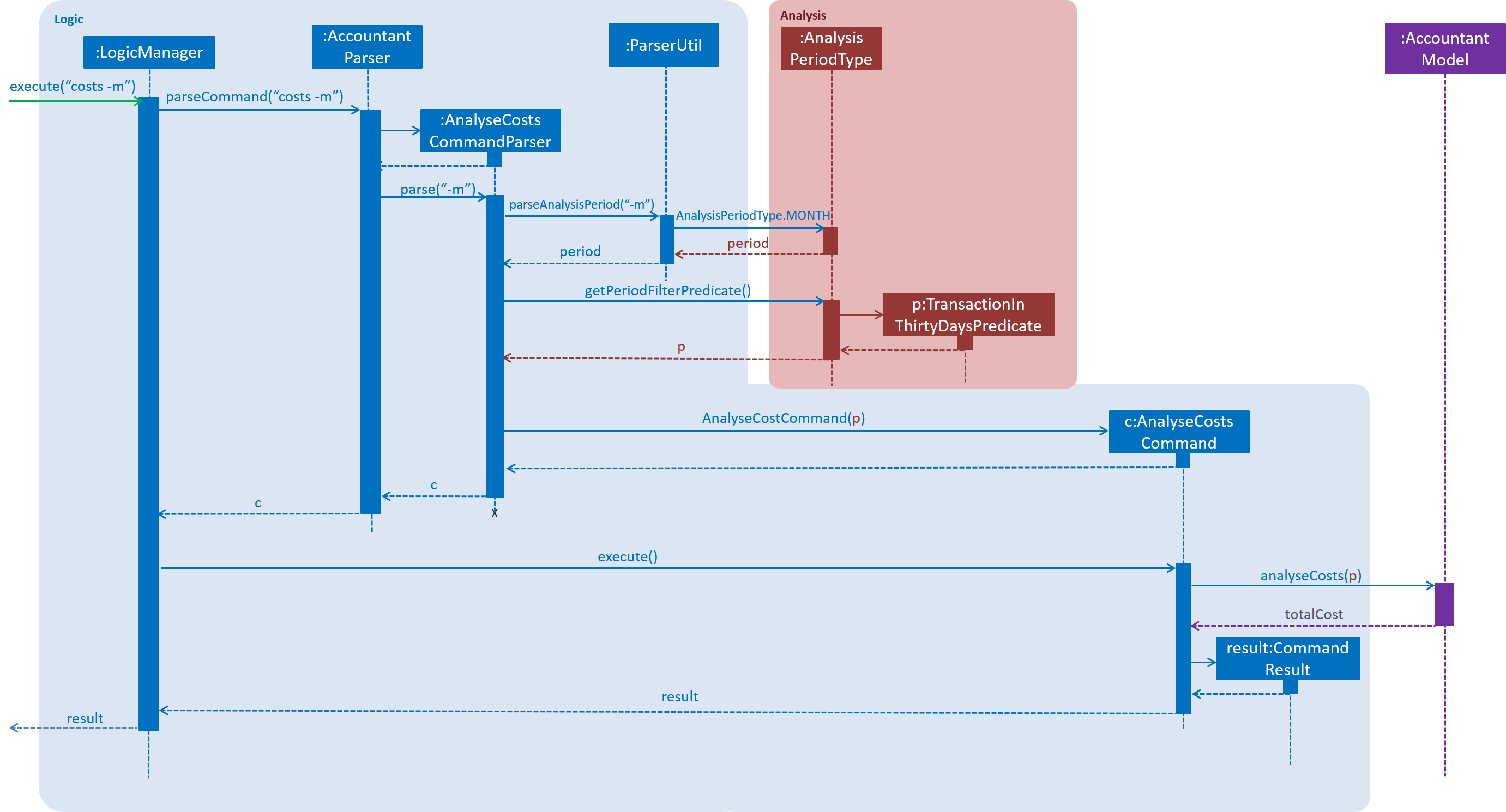
3.1.3. Implementation of Analysis#analyseCost()
The actual analysis of costs continues from the the filtering of the filtered transaction list. This section focuses on the actual computation of the costs incurred by the company.
Step 1. In addition to passing the analysis period Predicate, a PurchaseTransactionPredicate
is
also passed to the filtered transaction list simultaneously.
This filters the list further, leaving only the TransactionType.PURCHASE
transactions, which contribute
to the costs of the company.
Overall, the filtered transaction list will also be updated in the UI.
Step 2. ModelManager
passes control to the AnalysisManager
.
Step 3. In AnalysisManager
, the helper method AnalysisManager#calculateTotalCost()
is called
to compute the total costs of the filtered transactions.
It iterates through the filtered transaction list, and checks the Transaction
's TransactionType
as a precuation. If the TransactionType
is PURCHASE
, the amountMoney
transacted is obtained and
added to a sum of costs. The sum of costs is then wrapped in a Price
object and returned to the calling function.
3.1.4. Implementation of Analysis#analyseRevenue()
The implementation of the analysis of revenue is similar to that of costs. However, a SaleTransactionPredicate
is passed to the filtered transaction list instead.
In Step 3, the helper method AnalysisManager#calculateTotalRevenue()
is called. It iterates
through the filtered transaction list, and checks if the Transaction
's TransactionType
is
SALE
. If so, the amountMoney
transacted is added to the sum of revenues. The sum of revenues
is then wrapped in a Price
object and returned to the calling function.
3.1.5. Implementation of Analysis#analyseProfit()
The analysis of profit continues from the filtering of the filtered transaction list by period. This section focuses on the actual computation of the profit earned by the company.
Step 1. ModelManager
passes control to the AnalysisManager
. No additional filtering is done.
Step 2. In AnalysisManager
, the helper method AnalysisManager#calculateTotalProfit()
is called
to compute the total profit of the filtered transactions.
It iterates through the filtered transaction list, and checks the Transaction
's TransactionType
.
-
If the
TransactionType
isPURCHASE
, theamountMoney
transacted is obtained and added to a sum of costs. -
If the
TransactionType
isSALE
, theamountMoney
transacted is obtained and added to a sum of revenues.
Step 3. Total profit is calculated using the formula: "profit = revenue - cost". This total profit value is checked to determine whether it is negative.
-
If total profit is positive or zero, it is wrapped in a
Price
object and returned to the calling function. -
If the total profit is negative, the absolute value is taken, and a special
NegativePrice
object is used to wrap the value. It is then returned to the calling function.-
NegativePrice
is a sub-class ofPrice
, and is used only when calculating profit. -
The
value
of theNegativePrice
object is the absolute value of the profit.
-
3.1.6. Design Considerations
Aspect: Filtering the transactions by date
-
Current implementation: Pass predicates to the filtered transaction list, before performing analyses using that list
-
Pros:
-
Extensible as more Predicates can be created and passed to the filtered transaction list to filter the transactions by different facets.
-
Enhances reusability especially of the
updateFilteredTransactionList(Predicate predicate)
method. -
Allows the transaction list panel in the UI to be updated automatically.
-
-
Cons: We must ensure that the implementation of each Predicate is correct.
-
-
Alternative: Loop through all transactions in the
TransactionList
, and check if the transaction date is within the desired time period-
Pros: Easy to implement.
-
Cons: May have time performance issues if there are many transactions.
-
3.2. Command partition
3.2.1. Current Implementation
The command partition is an implementation of the role system.
The model
contain all the
API that is common for every user. StockTakerModel
contains API for for stockTaker. Similar
idea applied to AccountantModel
and ManagerModel
. However, AdminModel
extends all three models.
As such, adminModel
will contains all APIs.
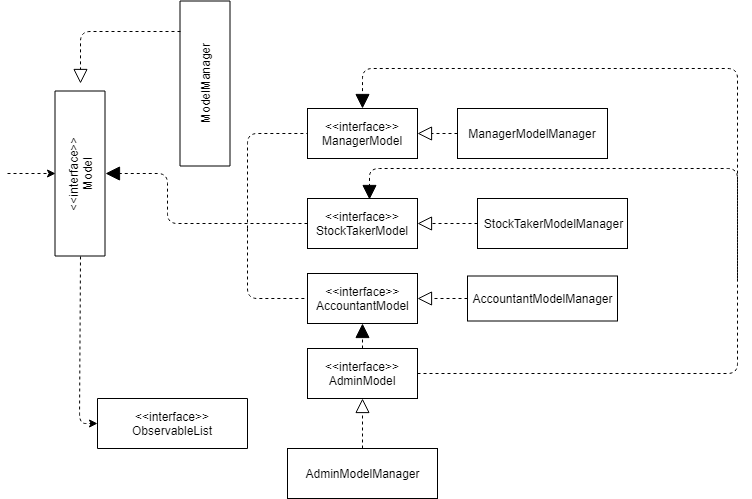
After login, logicManager
will assign a particular role to user according to their
authenticationLevel
. As such, it will prevent one role from accessing other role’s
command.
3.3. Login/logout feature
3.3.1. Current Implementation
The login feature is a standalone feature that enable security check on user. It has a fxml page that name LoginPage.fxml
at main\resources\view
The controller of the fxml page named LoginController
at seedu.address\controller
The model of is at loginInfo
which storage the format in JSON with the help of JsonUtils
.
Also, there is a loginInfoManager
which include all the API for loginInfo
.
As such, this is a design that fulfil the Model-View-Controller pattern.
Given below is a class diagram for login function. LoginUtils has attributes of LoginInfoModel
userName
and Password
. It also use passwordUtils
to hashed verify the password with LoginInfoModel
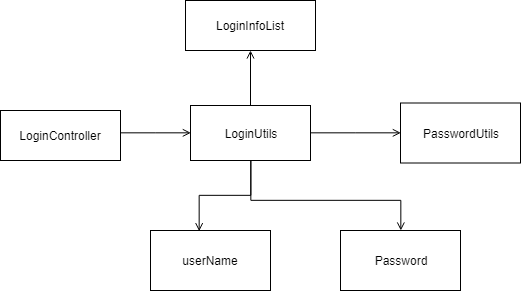
Given below is an example usage scenario and how login mechanism behave at each step.
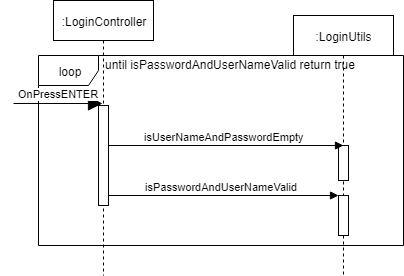
The LoginController
will check for username and password will the LoginUtils
.
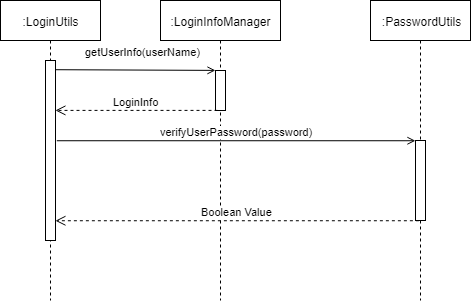
3.4. Save information about user account
Given below is a structure of Model
components that is related to login feature.
The model stores loginInfo of the user.
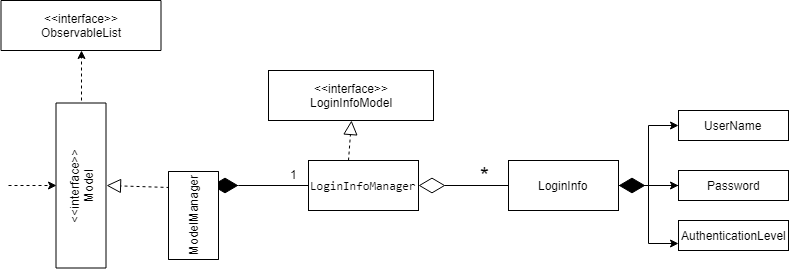
The sequence diagram below shows the interactions within the logic components for the execuion of createAccount
command.
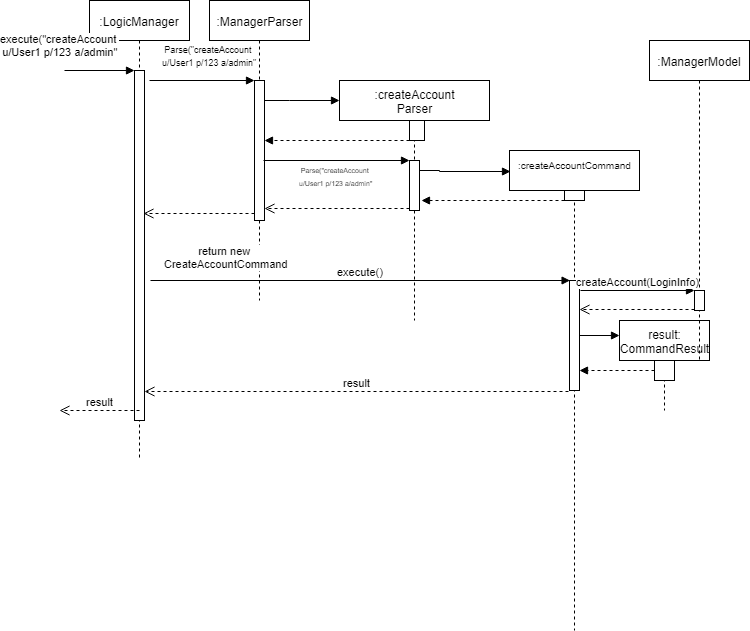
Given below is an sequence diagram of access login information for loginInfoList.json
during initiation of the application.
The program also save the the login information to loginInfoList.json
when logout
or exit
.
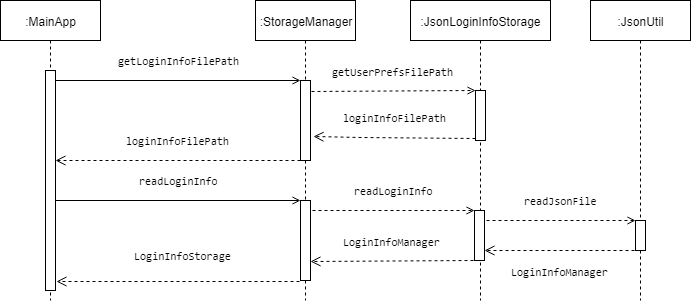
3.4.1. Design Considerations
Aspect: How to store the data
-
Alternative 1 (current choice): Saves the login detail in a json file called
loginInfoList.json
.-
Pros: Have a systematic and elegant way to store data.
-
Cons: Hard to implement
-
-
Alternative 2: Store the data in enum.
-
Pros: Easy to implement
-
Cons: Fixed database. Cannot add /modify/delete accounts. (suitable for very small project)
-
Aspect: Data format for store data
-
Alternative 1 (current choice): Store in Json file.
-
Pros: Json is popular and have many support online.
-
Pros :JSON is relatively easier to implement compared to XML
-
Cons: Have to write serialized method for JSON file.
-
-
Alternative 2: Store in XML file
-
Pros: Classic and matured product
-
Pros: Have serialized code in original ab4.
-
Cons: It has many rules to set before implementation.
-
3.5. Inventory Management
In Drink I/O, inventory is managed in the model, through the use of Drink Objects with the attributes name
, price
, quantity
, tags
, UniqueBatchList
. In Drink I/O, drink purchases are stored in Batch
objects which are made unique by their BatchDate
attribute.
These Batch
objects contain a BatchId
, a BatchQuantity
and a BatchDate
attribute and are stored in the UniqueBatchList
of the relevant drink.
3.5.1. Buying a drink with buy n/DRINK_NAME q/QUANTITY
A user may enter a buy command with the above parameters to record a purchase of drinks.
The following is the sequence of steps when the user enters an buy command with specified name and quantity:
Step 1. User enters a buy command (i.e. buy
n/Coca cola
q/400
).
Step 2. The appropriate parser parses the name and quantity parameters and assigns the name
and quantity
variables to the buy
command.
Step 3. The buy command is executed, and a new Transaction
object is created with the name
, quantity
and a transactionType
variable. This Transaction
object is then passed to the StockTakerModelManager.
Step 4. In the StockTakerModelManager, recordTransaction(Transaction)
is used to record the transaction and increaseDrinkQuantity(Name, Quantity)
is called to update the change in quantity in the inventory list.
Step 5. The inventory list then passes the name
and quantity
variables down to the UniqueDrinkList
which calls the increaseQuantity(Quantity)
method in the relevant Drink
object.
Step 6. In the Drink
object, a new Batch
object is created with the name
and quantity
variables and passed to the Drink’s UniqueBatchList
through the addBatch
method.
Step 7. In the UniqueBatchList
, the Batch
that was passed down would be checked against the other Batch
objects in the list for uniqueness based on its date. If it has a unique date, a new Batch
would be created and stored in the UniqueBatchList
, else the currently existing Batch
object in the UniqueBatchList
with the same date will have its Quantity
attribute incremented by the quantity
value of the Batch
object added.
Step 8. The totalQuantity
attribute of the UniqueBatchList
is then updated by looping through the batches in the list, and is handed up to the Drink
object which updates its Quantity
attribute with the value of the totalQuantity
attribute.
3.5.2. Selling a drink
To facilitate sales of drinks from the stock of the company, sales of drinks from the inventory are conducted with the command sell
n/DRINK_NAME
q/QUANTITY
A user may enter a buy command with the above parameters to record a purchase of drinks.
The following is the sequence of steps when the user enters an buy command with specified name and quantity:
Step 1. User enters a sell command (i.e. sell
n/Coca cola
q/400
).
Step 2. The appropriate parser parses the name and quantity parameters and assigns the name
and quantity
variables to the sell
command.
Step 3. The sell command is executed, and a new Transaction
object is created with the name
, quantity
and a transactionType
variable. This Transaction
object is then passed to the StockTakerModelManager.
Step 4. In the StockTakerModelManager, recordTransaction(Transaction)
is used to record the transaction and decreaseQuantity(Name, Quantity)
is called to update the change in quantity in the inventory list.
Step 5. The inventory list then passes the name
and quantity
variables down to the UniqueDrinkList
which calls the decreaseQuantity(Quantity)
method in the relevant Drink
object.
Step 6. In the Drink
object, updateBatchTransaction(Quantity)
is called and the quantity
variable is passed down to the UniqueBatchList
.
Step 7. In the UniqueBatchList
, quantity
passed down will be checked against the totalQuantity
variable. If quantity
is more than totalQuantity
, a InsufficientQuantityException will be thrown which would be handled in the SellDrinkCommand
class. Else, the updateBatchTransaction
would loop through the UniqueBatchList
and decrement the quantity of the individual batches starting from the one with the oldest date.
Step 8. The totalQuantity
attribute of the UniqueBatchList
is then updated by looping through the batches in the list, and is handed up to the Drink
object which updates its Quantity
attribute with the value of the totalQuantity
attribute.
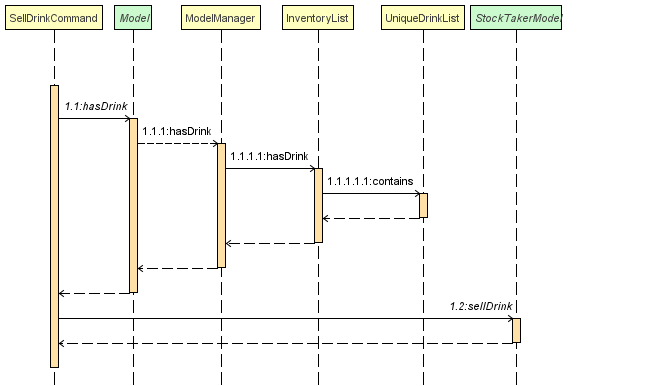
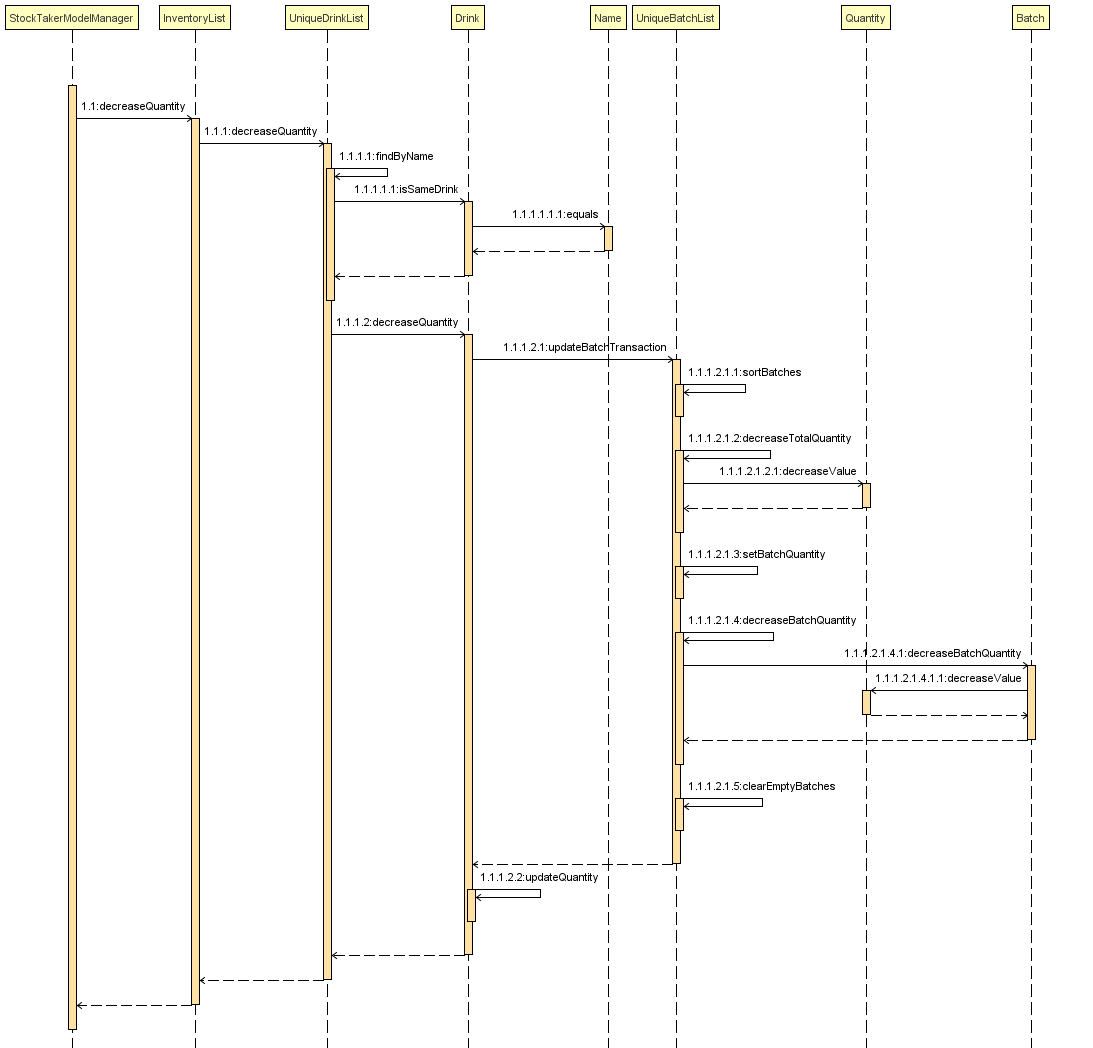
3.5.3. Design Considerations
Aspect: Facilitating the Buy and Sell Commands
-
Current Implementation: Sorting the
UniqueBatchList
byBatchDate
using a Custom Comparator.-
Pros:
-
Makes implementation of methods such as
addBatch
,updateBatchTransaction
,getEarliestBatchDate
,getOldestBatchDate
much easier asBatch
objects are already indate
order. -
Easy to modify, allowing users to sort batches based on
quantity
by just editing the Custom Comparator. -
Allows batches shown in the BatchListPanel to be easily ordered based on date.
-
-
Cons:
-
When users replace the Custom Comparator with another comparator, methods such as
getEarliestBatchDate
will have to be edited as they depend on theUniqueBatchList
being in date-sorted order.
-
-
-
Alternative: Loop through all batches in the
UniqueBatchList
for all methods.-
Pros: When the
UniqueBatchList
is sorted differently, methods do not have to be rewritten. -
Cons: May have time performance issues if there are many batches.
-
3.6. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 3.7, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
3.7. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file (default: config.json
).
4. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
4.1. Editing Documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
4.2. Publishing Documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
4.3. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
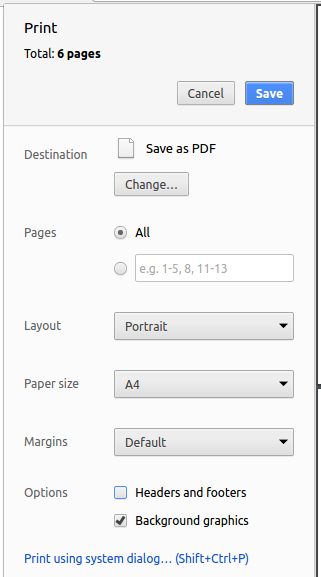
4.4. Site-wide Documentation Settings
The build.gradle
file specifies some project-specific asciidoc attributes which affects how all documentation files within this project are rendered.
Attributes left unset in the build.gradle file will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
The name of the website. If set, the name will be displayed near the top of the page. |
not set |
|
URL to the site’s repository on GitHub. Setting this will add a "View on GitHub" link in the navigation bar. |
not set |
|
Define this attribute if the project is an official SE-EDU project. This will render the SE-EDU navigation bar at the top of the page, and add some SE-EDU-specific navigation items. |
not set |
4.5. Per-file Documentation Settings
Each .adoc
file may also specify some file-specific asciidoc attributes which affects how the file is rendered.
Asciidoctor’s built-in attributes may be specified and used as well.
Attributes left unset in .adoc files will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
Site section that the document belongs to.
This will cause the associated item in the navigation bar to be highlighted.
One of: * Official SE-EDU projects only |
not set |
|
Set this attribute to remove the site navigation bar. |
not set |
4.6. Site Template
The files in docs/stylesheets
are the CSS stylesheets of the site.
You can modify them to change some properties of the site’s design.
The files in docs/templates
controls the rendering of .adoc
files into HTML5.
These template files are written in a mixture of Ruby and Slim.
Modifying the template files in |
5. Testing
5.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
5.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
seedu.address.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.address.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.address.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.address.logic.LogicManagerTest
-
5.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
HelpWindow.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
6. Dev Ops
6.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
6.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
6.3. Coverage Reporting
We use Coveralls to track the code coverage of our projects. See UsingCoveralls.adoc for more details.
6.4. Documentation Previews
When a pull request has changes to asciidoc files, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged. See UsingNetlify.adoc for more details.
6.5. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
6.6. Managing Dependencies
A project often depends on third-party libraries. For example, Address Book depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Product Scope
Target user profile:
-
has the need to manage a small drinks distribution company
-
prefer desktop apps over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: Manage a distribution company more conveniently than using paper or Excel, with the incorporation of inventory management and accounting tools in one app.
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
new user |
see usage instructions |
refer to instructions when I forget how to use the App |
|
Manager |
add a new drink item |
keep list of offerings updated |
|
Manager |
delete a drink item |
keep list of offerings updated |
|
Manager |
modify the price of a drink item |
maintain a good profit margin |
|
Manager |
see drink batches that have been imported before a certain date |
take action to clear old inventory stocks |
|
Manager |
see which drinks are selling well and the converse |
take action to manage stocks better |
|
Manager |
view actions done by employees |
track work done and monitor for fraud |
|
Stock Taker |
add new batches of drinks |
keep inventory updated |
|
Stock Taker |
update the stocks of drinks |
keep inventory updated |
|
Stock Taker |
view the stock numbers of drinks |
know when to restock them |
|
Stock Taker |
view import dates of drink batches |
sell the batches that have been in the inventory for longer |
|
Accountant |
update the cost price of drinks |
ensure that the total costs and profits of the company are updated |
|
Accountant |
keep track of the quantities of drinks sold over a certain period |
report on the trends on drink sales |
|
Accountant |
keep track of total profit over a period of time |
report on the status and progress of the company |
|
user |
type commands easily |
perform actions easily |
|
user |
login security feature |
secure important data |
|
user |
view list of available commands |
know which actions i can take |
|
user |
confirmation for actions |
reduce mistakes due to carelessness |
|
user |
see the pictures of drinks |
easily identify the drink that i am looking at |
|
experienced user |
have shortcuts for commands |
perform actions efficiently |
Appendix C: Use Cases
(For all use cases below, the System is the DrinkIO
and the Actor is the user
, unless specified otherwise)
Use case: Add Transaction
MSS
-
User requests to add specific item to sales list
-
System requests for confirmation
-
User confirms
-
System responds with successful add message
Use case ends.
Extensions
-
2a. User enters a non-existing item.
-
2a1. System responds that the item does not exist and requests for next command.
Use case ends.
-
-
2b. User enters a item that is already sold out
-
2b1. System responds that the item is sold out and requests for the next command.
Use case ends.
-
-
2c. User specified a quantity that is larger than the available stock
-
2c1. System prompts user to enter a valid sales number
Use case resumes from 1.
-
-
3a. User cancels procedure.
-
Use case ends.
-
Appendix D: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
9
or higher installed. -
Should be able to hold up to 1000 persons without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
Appendix F: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
F.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample drinks and transactions. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
F.2. Add a drink
-
Adding a drink into the inventory, using the
Manager
orAdmin
account-
Test case:
add n/Coca Cola Original2 p/20.00 cp/10.00 t/SoftDrink
Expected: Message displayed - "New drink added: Drink name: Coca Cola Original2 Cost price: 10.00 Selling price: 20.00, Tags: [SoftDrink]" -
Test case:
add n/Coca Cola Original2 p/20.00 cp/10.00 t/SoftDrink
(repetitive test)
Expected: Message displayed - "This drink already exists in the inventory list"
-
F.3. Delete a drink
-
Deleting a drink from the inventory, using the
Manager
orAdmin
account-
Prerequisites: There must be at least one drink in the inventory
-
Test case:
delete 1
Expected: Message displayed - "Deleted Drink:", followed by deleted drink’s details (name, cost price, selling price and tags) -
Test case:
delete 100000
Expected: Message displayed - "The drink index provided is invalid" -
Test case:
delete 0
Expected: Message displayed - "Invalid command format!"
-
F.4. Edit details of a drink
-
Editing a drink’s selling price, cost price or tags, using the
Manager
orAdmin
account-
Prerequisites: There must be at least one drink in the inventory
-
Test case:
edit 1 cp/400
Expected: Message displayed - "Edited Drink:", followed by edited drink’s details (name, cost price, selling price and tags) -
Test case:
edit 1 p/1
Expected: Message displayed - "Edited Drink:", followed by edited drink’s details (name, cost price, selling price and tags) -
Test case:
edit 1 t/PROMO
Expected: Message displayed - "Edited Drink:", followed by edited drink’s details (name, cost price, selling price and tags) -
Test case:
edit 1 cp/40 p/80
Expected: Message displayed - "Edited Drink:", followed by edited drink’s details (name, cost price, selling price and tags) -
Test case:
edit 1 cp/40 t/TAG1 t/TAG2
Expected: Message displayed - "Edited Drink:", followed by edited drink’s details (name, cost price, selling price and tags) -
Test case:
edit 1 cp/bla
Expected: Message displayed - "Price should contain only numbers and at most 1 decimal point. It must be least 1 digit long with a maximum of 2 digits after the decimal point" -
Test case:
edit 1 t/PROMO_TOO
Expected: Message displayed - "Tags names should be alphanumeric"
-
F.5. Buying a drink
-
Buy a certain quantity of a drink using the
buy
command, using aStock Taker
orAdmin
account-
Prerequisites: There must be at least one drink in the inventory, with one drink by the name of NAME.
-
Test case:
buy n/NAME q/12
Expected: The drink by the name of NAME (case-sensitive) has its quantity increased by 12. The message that "Purchase transaction recorded!" is shown in the results panel. -
Test case:
buy n/NAME q/12
, where NAME is the name of a drink that is not in the inventory
Expected: The message "The drink entered does not exist in the inventory list" is displayed.
-
F.6. Selling a drink
-
Sell a certain quantity of a drink using the
sell
command, using aStock Taker
orAdmin
account-
Prerequisites: There must be at least one drink in the inventory, with one drink by the name of NAME.
-
Test case:
sell n/NAME q/12
, where NAME is the name of a drink that is in the inventory, and its quantity is 12 or more.
Expected: The drink by the name of NAME (case-sensitive) has its quantity decreased by 12. The message that "Sale transaction recorded!" is shown in the results panel. -
Test case:
sell n/NAME q/12
, where NAME is the name of a drink that is not in the inventory
Expected: The message "The drink entered does not exist in the inventory list" is displayed. -
Test case:
sell n/NAME q/12
, where NAME is the name of a drink that is in the inventory, and its quantity is less than 12.
Expected: The message "Insufficient quantity in stock to perform operation" is displayed.
-
F.7. Analysing costs
-
Analyse costs over a period of a day, using an
Accountant
orAdmin
account-
Prerequisites: There must be at least one transaction in the transaction list.
-
Test case:
costs
Expected: The total cost incurred in a day is calculated and shown. ThePURCHASE
transactions are listed in the transaction list.
-
-
Analyse costs over a period of 7 days (including current day)
-
Prerequisites: There must be at least one transaction in the transaction list.
-
Test case:
costs -w
Expected: The total cost incurred in 7 days is calculated and shown. ThePURCHASE
transactions are listed in the transaction list.
-
-
Analyse costs over a period of 30 days (including current day)
-
Prerequisites: There must be at least one transaction in the transaction list.
-
Test case:
costs -m
Expected: The total cost incurred in 30 days is calculated and shown. ThePURCHASE
transactions are listed in the transaction list.
-
F.8. Analysing revenue
-
Analyse revenue over a period of a day, using an
Accountant
orAdmin
account-
Prerequisites: There must be at least one transaction in the transaction list.
-
Test case:
revenue
Expected: The total revenue earned in a day is calculated and shown. TheSALE
transactions are listed in the transaction list.
-
-
Analyse revenue over a period of 7 days (including current day)
-
Prerequisites: There must be at least one transaction in the transaction list.
-
Test case:
revenue -w
Expected: The total revenue earned in 7 days is calculated and shown. TheSALE
transactions are listed in the transaction list.
-
-
Analyse revenue over a period of 30 days (including current day)
-
Prerequisites: There must be at least one transaction in the transaction list.
-
Test case:
revenue -m
Expected: The total revenue earned in 30 days is calculated and shown. TheSALE
transactions are listed in the transaction list.
-
F.9. Analysing profit
-
Analyse profit over a period of a day, using an
Accountant
orAdmin
account-
Prerequisites: There must be at least one transaction in the transaction list.
-
Test case:
profit
Expected: The total profit earned in a day is calculated and shown. TheSALE
andPURCHASE
transactions within the time period are listed in the transaction list.
-
-
Analyse profit over a period of 7 days (including current day)
-
Prerequisites: There must be at least one transaction in the transaction list.
-
Test case:
profit -w
Expected: The total profit earned in 7 days is calculated and shown. TheSALE
andPURCHASE
transactions within the time period are listed in the transaction list.
-
-
Analyse profit over a period of 30 days (including current day)
-
Prerequisites: There must be at least one transaction in the transaction list.
-
Test case:
profit -m
Expected: The total profit earned in 30 days is calculated and shown. TheSALE
andPURCHASE
transactions within the time period are listed in the transaction list.
-
F.10. Changing password
-
Changing the password using
changePassword
-
Test case:
changePassword o/123 n/1234
(assuming 123 is the default old password)
Expected: Password has successfully changed to: 1234 -
Test case:
changePassword o/123xyz n/1234
(assuming 123 is the default old password)
Expected: The old password is wrong
-
F.11. Deleting account
-
Deleting an account, using the
Manager
orAdmin
account-
Test case:
deleteAccount u/manager
Expected:The account has been deleted -
Test case:
deleteAccount u/unknownAccount
Expected: This account does not exist
-